In this comprehensive tutorial, we will walk you through leveraging the powerful Pexels API to create your very own platform for accessing and showcasing a vast collection of high-quality, royalty-free images.
Table of Contents
Pexels API: An Overview
What is the Pexels API?
It’s a RESTful JSON API that helps developers access the Pexels content library(photos and videos).
Pexels offer’s this API to enable seamless integration of their media library into various applications and websites.
The API allows search for, retrieve, and display of stunning visuals. All photos and videos on Pexels are free to use.
Key features & benefits of using the Pexels API
- Vast and Diverse Media Collection
- High-Quality Visuals
- User-Friendly Documentation
- Free and Commercial Use
- Powerful Search Functionality
- Responsive Support
How the Pexels API works
Integrating the Pexels API into your stock photos website involves making HTTP requests to the API’s endpoints and handling the JSON responses returned. These responses contain essential information about each media item, such as image URLs, photographer details, video files, etc.
Rate limits
Pexels API sets rate limits to prevent abuse, maintain performance, and ensure a consistent user experience. By default, the API is rate-limited to 200 requests per hour and 20,000 requests per month.
Usage Guidelines
Display a link to Pexels whenever you make an API call. You can use their logo or a text link (such as “Photos provided by Pexels”).
Whenever possible, give proper credit to the photographers (for instance, “Photo by John Doe on Pexels” with a link to the image’s page on Pexels).
Setting up the Development Environment
To create and run our application, first, we need to set up our system with the following.
- Local Server:- XAMPP
- Code Editor:- whichever you like ( we are using VS code)
- Programming language:- You can use any programing language/framework. (we are going to use PHP)
Registering for Pexels API Key
– Signing up for a Pexels API account.
To get the API key, register and create an account on Pexels. Use this link(https://www.pexels.com/login/) to register yourself.
– Obtaining the API key for authentication.
Now that you have created an account, go to their menu and look for- Photo & Video API.
To know, how to register for Pexels API, check out this video – How to get your Pexels API Key.
Making API Requests: Retrieving Free Stock Photos
<!DOCTYPE html> <html> <head> <title>Pexels API Example</title> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> </head> <body> <form method="post"> <label for="search">Search:</label> <input type="text" id="search" name="search"> <label for="per_page">Results:</label> <input type="number" id="per_page" name="per_page" min="1" max="100" value="10"> <button type="submit">Submit</button> </form> </body> </html>

<?phpif ($_SERVER['REQUEST_METHOD'] === 'POST') {// Pexels API endpoint URL$url = "https://api.pexels.com/v1/search";// Pexels API key$api_key = "YOUR-API-KEY";// Retrieve the search query and number of results from the POST request$query = $_POST['search'];$per_page = $_POST['per_page'];// Set up cURL$ch = curl_init();curl_setopt($ch, CURLOPT_URL, $url . "?query=" . urlencode($query) . "&per_page=" . $per_page);curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);curl_setopt($ch, CURLOPT_HTTPHEADER, array("Authorization: " . $api_key));// Execute the API request and fetch response$response = curl_exec($ch);curl_close($ch);// Parse the response as JSON$photos = json_decode($response, true);// Print the list of photosforeach ($photos["photos"] as $photo) {echo $photo["id"] . ": " . $photo["src"]["medium"] . "<br>";}}?>
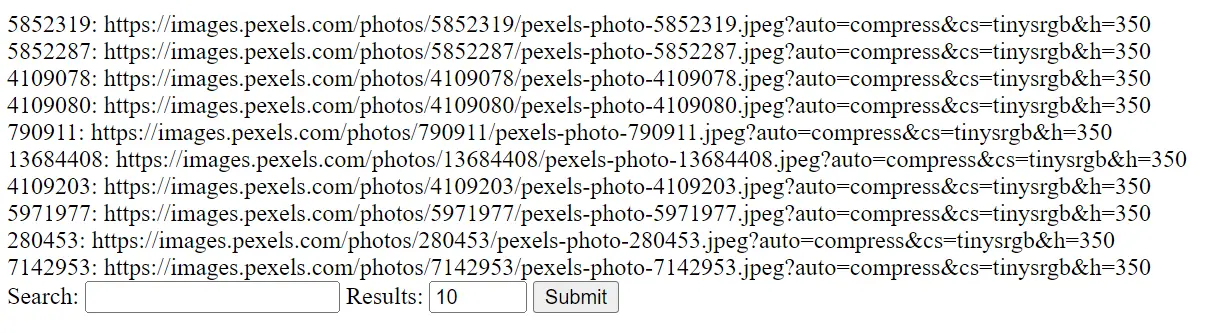
Step 1: Request Method Check
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
This line checks if the current request method is POST. It ensures that the code inside this block is only executed when the form is submitted using the POST method.
Step 2: API Endpoint and API Key
$url = "https://api.pexels.com/v1/search"; $api_key = "pexels-api-key";
Here, we define the API endpoint URL (https://api.pexels.com/v1/search) provided by Pexels. It’s the URL that we will use to make the API request. Additionally, we store the API key in a variable. This key is required to authenticate and authorize the API request.
Step 3: Retrieve Search Query and Results Per Page
$query = $_POST['search']; $per_page = $_POST['per_page'];
These lines retrieve the search query and the number of results per page from the submitted form data. The “$_POST” superglobal array is used to access the values submitted via the POST request.
Step 4: cURL Setup
$ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url . "?query=" . urlencode($query) . "&per_page=" . $per_page); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_HTTPHEADER, array( "Authorization: " . $api_key ));
In this step, we set up the cURL library to make the API request. curl_init() initializes a cURL session. We then set various options using curl_setopt().
- CURLOPT_URL is set to the API endpoint URL with the search query and number of results per page added as query parameters. The urlencode() function is used to properly encode the search query.
- CURLOPT_RETURNTRANSFER is set to true to ensure that the API response is returned as a string instead of being directly outputted.
- CURLOPT_HTTPHEADER is set to include the Authorization header with the API key.
$response = curl_exec($ch); curl_close($ch);Here, we execute the cURL request using “curl_exec()“. This sends the request to the Pexels API and fetches the response. We store the response in the $response variable. Finally, we close the cURL session using “curl_close()“.
Step 6: Parse API Response
$photos = json_decode($response, true);
We parse the API response, which is in JSON format, into a PHP associative array using “json_decode()“. The “true” parameter specifies that we want an associative array instead of an object.
Step 7: Display the List of Photos
foreach ($photos["photos"] as $photo) { echo $photo["id"] . ": " . $photo["src"]["medium"] . "||" "; }
In this step, we iterate through the array of photos obtained from the API response. For each photo, we echo its ID and the URL of the medium-sized image. This code snippet is a basic example of how you can display the retrieved photos, but you can modify it to suit your specific needs.
Making API Requests: Retrieving Free Stock Videos
Add the bellow code:-
// Pexels API endpoint URL $url = "https://api.pexels.com/videos/search";
In this modified version, we have updated the API endpoint URL to point to the video search endpoint (https://api.pexels.com/videos/search).
Add the bellow code:-
// Parse the response as JSON $videos = json_decode($response, true); // Print the list of videos foreach ($videos["videos"] as $video) { echo $video["id"] . ": " . $video["video_files"][0]["link"] . " "; }
The response parsing and printing section remain the same, but we are now iterating through the “videos” array instead of the “photos” array. Additionally, we are printing the video’s ID and the URL of the first video file in the “video_files” array.
With this code, you can make a video request to the Pexels API and display the list of videos obtained from the response.
Building the Image Gallery
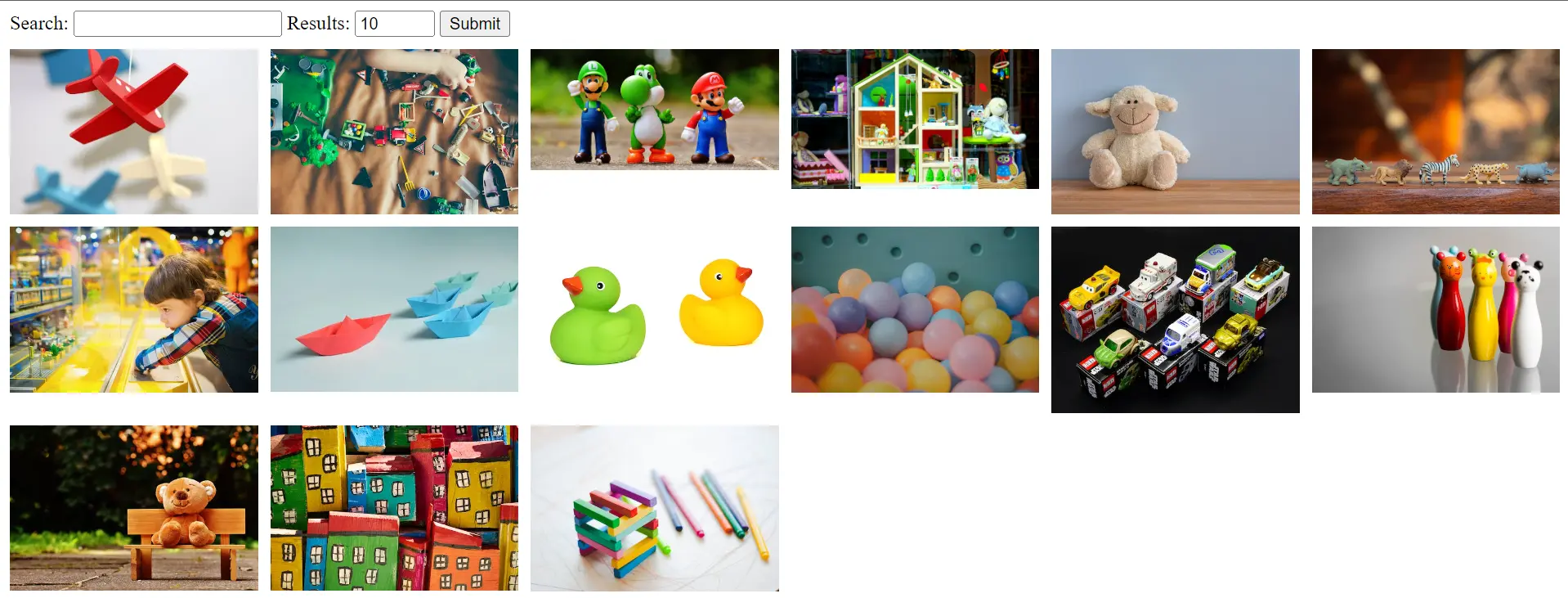
Let’s understand the code step by step.
- The “isset($photos)” condition checks if the “$photos” variable is set and not null. It ensures that we have received a valid response from the Pexels API and that the “$photos” array contains data.
- The “$photos[“total_results”] > 0” condition checks if there are any results available in the “$photos” array. If there are no results, the code will execute the “else” block and display the “No results found” message.
- If there are results available, the code will execute the if block. It starts by echoing an opening “div” tag with the photo-grid CSS class. This sets up a grid layout to display the images.
- Inside the foreach loop, the code iterates over each photo in the “$photos[“photos”]” array. For each photo, it echoes an
tag with the src attribute set to the medium-sized image URL “($photo[“src”][“medium”])“. The alt attribute is set to the photo’s alt text “($photo[“alt”])“.
- After iterating through all the photos, the code echoes a closing “div” tag to close the grid container.
- If there are no results “($photos[“total_results”] is 0)“, the code will execute the else block and display a “P” tag with the “No results found” message.
In summary, this code checks if there are photos available in the “$photos” array and dynamically generates HTML code to display the photos in a grid layout. If no results are found, it displays a message indicating the absence of results.
Conclusion
Congratulations! You have successfully learned how to build your own free stock photos website using the Pexels API. By leveraging the Pexels API’s capabilities and creating an engaging platform for users to access and utilize a wide range of high-quality, royalty-free images.
So, go ahead and start building your unique free stock photos website today!